Chapter 0
Setting Up Brainfuck
Table of Contents
Brainfuck was designed to be easily interpretable and lightweight. This makes getting set up very simple. There are a number of options to set up a Brainfuck ecosystem, some of which are discussed here.
0.1: Repl.it
repl.it is a free, online, and collaborative IDE that supports a wide number of languages, including Brainfuck (and many other esoteric languages). To get started, sign up for an account and create a Brainfuck repl:
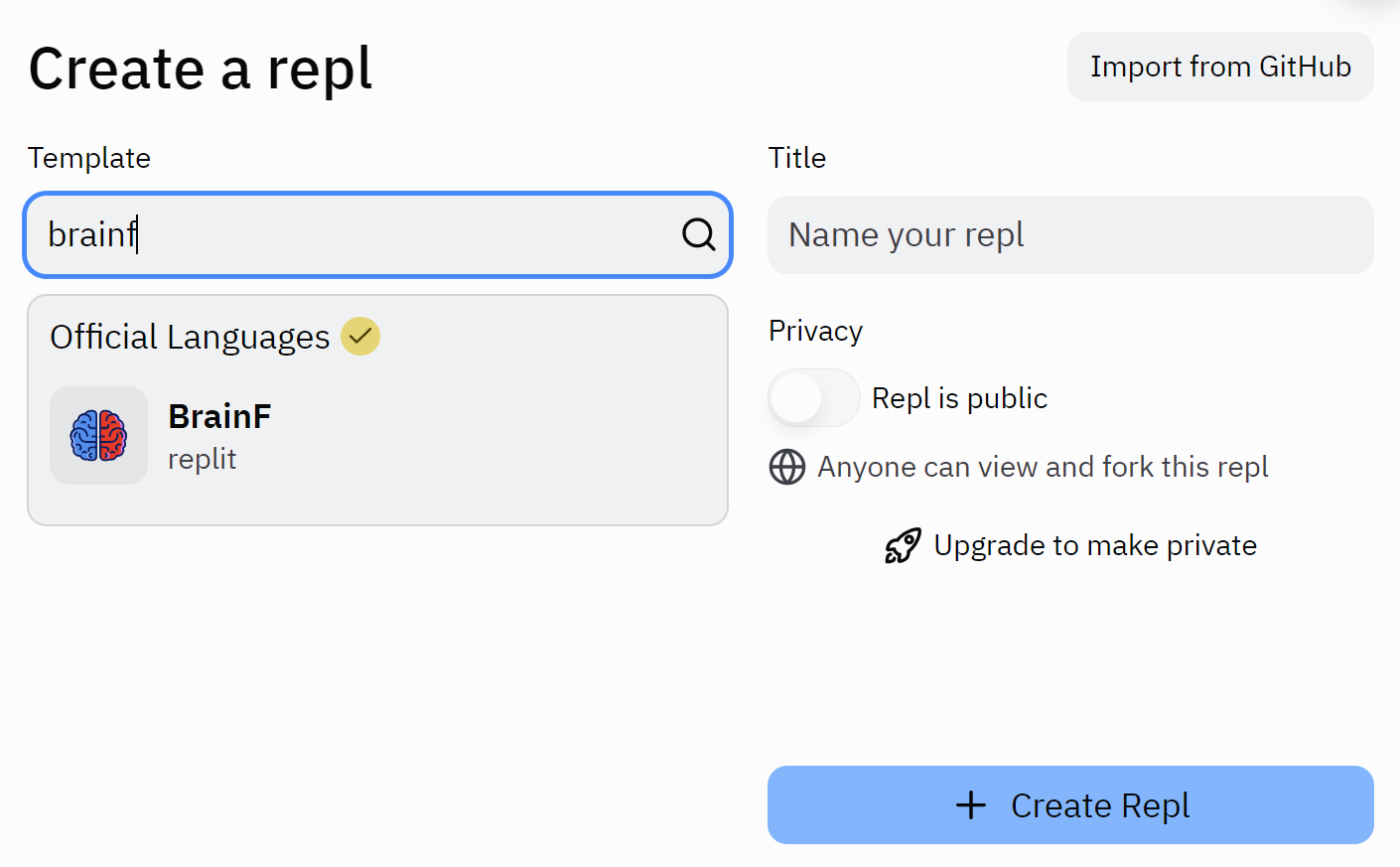
After you give the repl a name and click Create
, an editor will open. By default, repl.it opens to a Brainfuck implementation that prints out the Fibonacci series.
repl.it provides a set of helpful code ‘recipes’, which can be accessed by clearing all code in the .bf
file and clicking on the hyperlinked examples. Using repl.it is an easy way to get up and running with Brainfuck, especially if you want to collaborate.
0.2: Brainfuck Visualizers
Developing in Brainfuck can be significantly easier if code or code blocks are created with a visual reference of the digital tape being manipulated. There are a number of great Brainfuck visualizers, but this one and this one are probably some of the best. Users can enter Brainfuck code in a natural editor and the visualizers will highlight how your code manipulates values in the tape at each timestep.
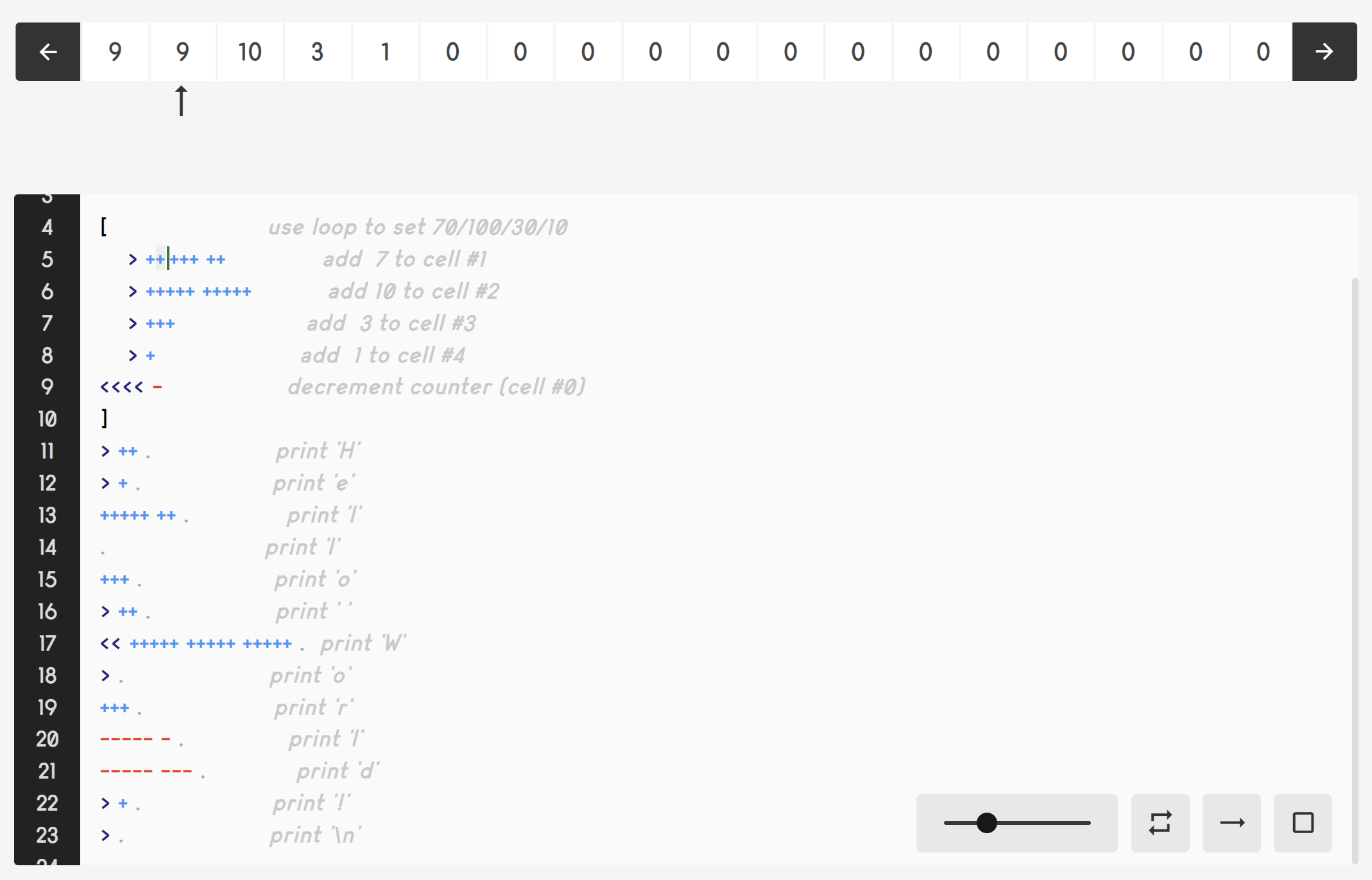
0.2: Online Interpreters
There are a number of online Brainfuck interpreters that give you instant output (as opposed to visualizing the output with a time delay):
0.3: Offline Interpreters
There is also software available to run Brainfuck offline:
0.4: DIY Interpreter
Brainfuck is perhaps one of the easiest languages to create an interpreter for. A programmer with intermediate experience can create an interpreter for Brainfuck code very quickly. If you’re feeling up to the task, it is not difficult to do it yourself. Below are some sample interpreters that people have written in different languages.
Python:
# Copyright 2011 Sebastian Kaspari
# From https://github.com/pocmo/Python-Brainfuck/blob/master/brainfuck.py
import sys
import getch
def execute(filename):
f = open(filename, "r")
evaluate(f.read())
f.close()
def evaluate(code):
code = cleanup(list(code))
bracemap = buildbracemap(code)
cells, codeptr, cellptr = [0], 0, 0
while codeptr < len(code):
command = code[codeptr]
if command == ">":
cellptr += 1
if cellptr == len(cells): cells.append(0)
if command == "<":
cellptr = 0 if cellptr <= 0 else cellptr - 1
if command == "+":
cells[cellptr] = cells[cellptr] + 1 if cells[cellptr] < 255 else 0
if command == "-":
cells[cellptr] = cells[cellptr] - 1 if cells[cellptr] > 0 else 255
if command == "[" and cells[cellptr] == 0: codeptr = bracemap[codeptr]
if command == "]" and cells[cellptr] != 0: codeptr = bracemap[codeptr]
if command == ".": sys.stdout.write(chr(cells[cellptr]))
if command == ",": cells[cellptr] = ord(getch.getch())
codeptr += 1
def cleanup(code):
return ''.join(filter(lambda x: x in ['.', ',', '[', ']', '<', '>', '+', '-'], code))
def buildbracemap(code):
temp_bracestack, bracemap = [], {}
for position, command in enumerate(code):
if command == "[": temp_bracestack.append(position)
if command == "]":
start = temp_bracestack.pop()
bracemap[start] = position
bracemap[position] = start
return bracemap
def main():
if len(sys.argv) == 2: execute(sys.argv[1])
else: print("Usage:", sys.argv[0], "filename")
if __name__ == "__main__": main()
C++:
// From https://gist.github.com/migimunz/964338/4fd5c9ddfc477c4fb323848e137e48fafca423fc
#include <stdio.h>
#include <vector>
#include <iterator>
#include <sstream>
#include <algorithm>
#include <iostream>
#include <fstream>
#include <ctype.h>
//typedefs
typedef char WORD;
typedef std::vector<WORD> instruction_t;
typedef instruction_t::iterator instruction_pointer;
typedef std::vector<WORD> memory_t;
typedef memory_t::iterator memory_pointer;
//structs
struct environment
{
memory_t memory;
instruction_t instructions;
instruction_pointer ip;
memory_pointer mp;
environment() : memory(3000)
{
clear();
mp = memory.begin();
}
void clear()
{
instructions.clear();
ip = instructions.begin();
}
};
//prototypes
void interpret(environment &env);
int from_line(environment &env, std::string &line, int open_brackets = 0);
int from_stream(environment &env, std::istream &stream, int open_brackets = 0);
void interactive_mode(environment &env);
void print_word(WORD word);
int main(int argc, char** argv)
{
environment env;
//if no args, read from stdin. else, read from file specified as first argument
if(argc == 1)
{
interactive_mode(env);
}
else
{
std::ifstream stream = std::ifstream(argv[1]);
if(stream.is_open())
{
int open_brackets = from_stream(env, stream);
if(open_brackets == 0)
interpret(env);
else
std::cout << "Unmatched brackets!" << std::endl;
}
else
{
std::cout << "File not found : " << argv[1] << std::endl;
}
}
return 0;
}
void print_word(WORD word)
{
if(isprint(word))
std::cout << word;
else
std::cout << "0x" << std::hex << (int)word << std::dec;
}
int from_line(environment &env, std::string &line, int open_brackets)
{
std::istringstream stream = std::istringstream(line);
return from_stream(env, stream, open_brackets);
}
int from_stream(environment &env, std::istream &stream, int open_brackets)
{
if(open_brackets == 0)
env.instructions.push_back('\0');
WORD word;
while(true)
{
stream >> word;
if(!stream) break;
switch(word)
{
case '<': case '>': case '+': case '-': case ',': case '.':
env.instructions.push_back(word);
break;
case '[':
open_brackets++;
env.instructions.push_back(word);
break;
case ']':
open_brackets--;
env.instructions.push_back(word);
break;
default:
break;
}
}
if(open_brackets == 0)
env.instructions.push_back('\0');
return open_brackets;
}
void interactive_mode(environment &env)
{
int open_brackets = 0;
while(true)
{
std::string line;
std::getline(std::cin, line);
if(!std::cin) break;
if(open_brackets == 0) env.clear();
open_brackets = from_line(env, line, open_brackets);
if(open_brackets == 0)
{
interpret(env);
std::cout << ">";
print_word(*env.mp);
std::cout << std::endl;
}
else
{
std::cout << "->";
}
}
}
void find_closing(environment &env)
{
int balance = 1;
do
{
env.ip++;
if(*env.ip == '[')
balance++;
else if(*env.ip == ']')
balance--;
}while(balance != 0);
}
void find_opening(environment &env)
{
int balance = 0;
do
{
if(*env.ip == '[')
balance++;
else if(*env.ip == ']')
balance--;
env.ip--;
}while(balance != 0);
}
void interpret(environment &env)
{
env.ip = env.instructions.begin();
while(env.ip != env.instructions.end())
{
switch(*env.ip)
{
case '+':
(*env.mp)++;
env.ip++;
break;
case '-':
(*env.mp)--;
env.ip++;
break;
case '>':
if(env.mp != (env.memory.end()--))
env.mp++;
env.ip++;
break;
case '<':
if(env.mp != env.memory.begin())
env.mp--;
env.ip++;
break;
case '.':
print_word(*env.mp);
env.ip++;
break;
case ',':
WORD word;
std::cin >> word;
(*env.mp) = word;
env.ip++;
break;
case '[':
if(!(*env.mp))
find_closing(env);
env.ip++;
break;
case ']':
find_opening(env);
env.ip++;
break;
case '\0':
env.ip++;
break;
}
}
}
Brainfuck (a Brainfuck compiler written in Brainfuck):
From https://github.com/canoon/bfbf/blob/master/bf.bf
[-]+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<[>[-],>[-]+++++++++++++++++++++++++++++++++>>>[-]>>[-
]<<<<<<[->>>>+>>+<<<<<<]>>>>>>[-<<<<<<+>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<<]>>>>>[
-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->>+>
+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]>>>
[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-]>[
-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<]>>[-
<<+>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>[->>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>[->>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>[-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>
>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<
]<>>]<>>>>>[-]<<[->>+<<]<<<>>[-<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<
<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>
]<<<<<[->>>>>+<<<<<]>>]<<<<<<<+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[
-]]<<]>[-]>>>[-]<<[-]>>>[-]<<<<<[-]+>>>>>>>>[-]>>[-]<<<<<<<<<[->>>>>>>+>>+<<<<<<
<<<]>>>>>>>>>[-<<<<<<<<<+>>>>>>>>>]<[-]>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>[-<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<]<<<[-]+[[-]>>>[-]>[-]<<<[-
>>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<
[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->
+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<<<<[-]>>>>
>>>[-]]<<<<<<<[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<[->>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<+<<<]>>>[-<<<+>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]>[-
]<<<[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<+<<<<<]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<+<<<<<]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>[-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<
<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<
[->+<]<>>]<>>>>>[-<+<+>>]<[->+<]<<<<>>[-<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->
>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<
+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<[-]>>>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>>>+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[->>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<][-]+++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<
<<<<<<<[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<
<<<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-
]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>
+>+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-
]]<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<+>>>>[-]]<<[-]+++++++++++++++++++++++++++
++++++++++++++++++>>>[-]>>[-]<<<<<<<<[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>
>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->
>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[
-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+
>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<->>>>[-]]<<
[-]++++++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<<<<<<<<[->>>>>>+>>+
<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<<]>>>>>[-<<<<<+>>
>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->>+>+<<<]>>>[
-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>
]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-]>[-]<<[->+>
+<<]>>[-<<+>>]<[<<<<.>>>>[-]]<<[-]++++++++++++++++++++++++++++++++++++++++++++>>
>[-]>>[-]<<<<<<<<[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[
->>>>+>+<<<<<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>
]<[>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>
[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<
<[-]>>>>[-]]<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-],>>>>[-]]<<[-]++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<<<<<<<<[->>>>>>+>>+<
<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<<]>>>>>[-<<<<<+>>>
>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->>+>+<<<]>>>[-
<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]
<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-]>[-]<<[->+>+
<<]>>[-<<+>>]<[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<]>>
>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<<<]>>>
>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<
<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-
<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->
+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>]<>>>>>[-<<
<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-]<<[->>+<<]<<<<>>[-<+>]>[-<+>]>[-
<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>[-<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<
<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-
<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<]>>>>>[-<<
<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]>[-]<<<[-]<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<<
<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>[-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<
]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>]<>>>>
>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<+<+>>]<[->+<]<<<<<>>[-<+>]>
[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>[-<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<
[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>
[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<[-]>>>>>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>>>>>+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[->>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<]<[-]]<<[-]++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
++>>>[-]>>[-]<<<<<<<<[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<
<<<[->>>>+>+<<<<<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<
+>>>]<[>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>
[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<
[<<<<[-]>>>>[-]]<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[
->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<[-
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<
<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+
<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<
[->+<]<[->+<]<[->+<]<>>]<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[
-]<<[->>+<<]<<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>[-<<<>>[-<+>]>
[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>
>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<+<<<<<]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>[-]>[-]<<<[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<
<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
[-]<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]
<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[-
>+<]<[->+<]<[->+<]<[->+<]<>>]<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>
>>>>[-<+<+>>]<[->+<]<<<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>[-<<<
>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<
<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]>>>>>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>[-<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>
>>>>+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<]<[-]]<<[-]++++++++++++++++++++++++
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<<
<<<<<<[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<
<<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]
<<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+
>+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]
]<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[>[-]>[-]<<<<<<[->>>>>+>+<<<<<<]>>>>>>[-<<<<<<+
>>>>>>]<[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<<<<<]
>>>>>>>>>[-<<<<<<<<<+>>>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<
]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<<<<]>>>>>[
-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<>>>>>[-<<<<<+>>>>>
]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>
>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>]<>>>>>[-<<<<<+>>>>>]<[->+<]<[
->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-]<<
[->>+<<]<<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<
+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>[-<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<
<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<
+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>>
>>[-]][-]+>[-]>[-]<<<<<<<[->>>>>>+>+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]<[<[-]>[-]]
[-]>[-]<<[->+>+<<]>>[-<<+>>]<[>[-]+[<<<<<<<<<<+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<[->>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<+<]>[-<+>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]>[-]<<<[-]<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<
<<<<<<<<<<]>>>>>>>>>>>>[-<<<<<<<<<<<<+>>>>>>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<<<<<<[->
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<+<<<<<<<<<<<<]>>>>>>>>>>>>[-<<<<<<<<<<<<+>>>>>>>>>>>>]>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]
<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[-
>+<]<[->+<]<[->+<]<[->+<]<>>]<>>>>>[-<+<+>>]<[->+<]<<<<>>[-<<<>>[-<+>]>[-<+>]>[-
<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<
>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<[-]>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<][-]+++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++>>
>[-]>>[-]<<<<<<[->>>>+>>+<<<<<<]>>>>>>[-<<<<<<+>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<
<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<
<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>
+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]
<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<+>>>>[-]]<<[-]+++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<<<<<
<[->>>>+>>+<<<<<<]>>>>>>[-<<<<<<+>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<<]>>>>>[-<<<<<
+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->>+>+<<<]>
>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+
>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-]>[-]<<[-
>+>+<<]>>[-<<+>>]<[<<<<->>>>[-]]<<<<]<[-]]<<[-]]<<[-]+++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<<<
<<<<<[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<
<]>>>>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<
<<[->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>
+<<<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]
<<<[-]>[-]<<[->+>+<<]>>[-<<+>>]<[>[-]>[-]<<<<<<[->>>>>+>+<<<<<<]>>>>>>[-<<<<<<+>
>>>>>]<[<<<<->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-]<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+<<
<<<<<<<]>>>>>>>>>[-<<<<<<<<<+>>>>>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>[-]>[-]<<<[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<+<<<<<]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>[-]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<[-]<<<<<[->>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<+<<<<<]>>>>>[-<<<<<+>>>>>]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[
-<>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[-
>+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>]<>>>>>[-<
<<<<+>>>>>]<[->+<]<[->+<]<[->+<]<[->+<]<>>>>>>[-<<<<<+>>>>>]<[->+<]<[->+<]<[->+<
]<[->+<]<>>>>>>[-<+<+>>]<[->+<]<<<<<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<
<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>[-<<<>>[-<+>]>[-<+>]>[-<+
>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]<>>
[-<+>]>[-<+>]>[-<+>]>[-<+>]<<<<<[->>>>>+<<<<<]>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<[-]>>>>>>>>>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>[-<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<+>>>>>>>>>+>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<]<<<<<+>>>>[-]][-]+>[-]>[-]<<<<<<<[->>>>>>+>+<
<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>]<[<[-]>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<<->
>>>>[-]]<<[-]]<<[-]++++++++++++++++++++++++++++++++++++++++++>>>[-]>>[-]<<<<<<<<
[->>>>>>+>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]>[-]<<<<<[->>>>+>+<<<<<]>>>
>>[-<<<<<+>>>>>]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[->
>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<<]
>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<[-
]>[-]<<[->+>+<<]>>[-<<+>>]<[>>>>[-]<<[-]++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++>[-]>>>[-]>>[-]<<<<<
<<<<<<<[->>>>>>>>>>+>>+<<<<<<<<<<<<]>>>>>>>>>>>>[-<<<<<<<<<<<<+>>>>>>>>>>>>]<<<[
-]>>>[-]<<<<<<[->>>+>>>+<<<<<<]>>>>>>[-<<<<<<+>>>>>>]<<[>[-][-]>[-]>[-]<<<[->>+>
+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<<<[->>>>+>+<<<<<]>>>>>[-<<<<<+>>>>>]<[<<[-]+>>[-]
]<[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<-<->>>[-]]<[-]+>[-]>[-]<<<<[->>>+>+<<<<]>>
>>[-<<<<+>>>>]<[<[-]>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[>[-]>[-]<<<<[->>>+>+<<<<]
>>>>[-<<<<+>>>>]<[<<<<[-]>>>>>[-]<<<<<<<<[->>>+>>>>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<
+>>>>>>>>]<[-]]<<<<<<+>>>>>[-]]<<]>>[-]>[-]<<<<[->>>+>+<<<<]>>>>[-<<<<+>>>>]<[<<
[-]>>>[-]<<<<[->+>>>+<<<<]>>>>[-<<<<+>>>>]<<<<[-]>>>>[-]<<<<<<<[->>>+>>>>+<<<<<<
<]>>>>>>>[-<<<<<<<+>>>>>>>][-]<<<[-<->>>>+<<<]>>>[-<<<+>>>]<[-]]<<[-]>[-]<<<<[->
>>+>+<<<<]>>>>[-<<<<+>>>>]<[>[-]++++++++++++++++++++++++++++++++++++++++++++++++
>[-]<<<<<[->>>>+>+<<<<<]>>>>>[-<<<<<+>>>>>]<.<<<[-]+>>[-]]<<<<[-]++++++++++>[-]>
>>[-]>>[-]<<<[->+>>+<<<]>>>[-<<<+>>>]<<<<<<<[-]>>>>>>>[-]<<<<<<[-<+>>>>>>>+<<<<<
<]>>>>>>[-<<<<<<+>>>>>>]<<[>[-][-]>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<
<<<<<<<<[->>>>>>>>+>+<<<<<<<<<]>>>>>>>>>[-<<<<<<<<<+>>>>>>>>>]<[<<[-]+>>[-]]<[-]
][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<-<<<<<->>>>>>>[-]]<[-]+>[-]>[-]<<<<<<<<[->>>>>>
>+>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[<[-]>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]
<[>[-]>[-]<<<<[->>>+>+<<<<]>>>>[-<<<<+>>>>]<[<<<<<<<<[-]>>>>>>>>>[-]<<<<<<<<[-<+
>>>>>>>>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[-]]<<<<<<+>>>>>[-]]<<]>>[-]>[-]
<<<<<<<<[->>>>>>>+>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<[<<[-]>>>[-]<<<<<<<<[-
>>>>>+>>>+<<<<<<<<]>>>>>>>>[-<<<<<<<<+>>>>>>>>]<<<<<<<<[-]>>>>>>>>[-]<<<<<<<[-<+
>>>>>>>>+<<<<<<<]>>>>>>>[-<<<<<<<+>>>>>>>][-]<<<[-<<<<<->>>>>>>>+<<<]>>>[-<<<+>>
>]<[-]]<<[-]>[-]<<<<[->>>+>+<<<<]>>>>[-<<<<+>>>>]<[<<[-]+>>[-]][-]>[-]<<<[->>+>+
<<<]>>>[-<<<+>>>]<[>[-]++++++++++++++++++++++++++++++++++++++++++++++++>[-]<<<<<
[->>>>+>+<<<<<]>>>>>[-<<<<<+>>>>>]<.<[-]][-]++++++++++++++++++++++++++++++++++++
++++++++++++>[-]<<<<<<[->>>>>+>+<<<<<<]>>>>>>[-<<<<<<+>>>>>>]<.<<<<<<[-]]<<<<<<<
+>>>>>>>[-]>>[-]<<<<<<<<<[->>>>>>>+>>+<<<<<<<<<]>>>>>>>>>[-<<<<<<<<<+>>>>>>>>>]<
[-]>[-]>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>[-<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<+>+>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>]<<<<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<[->>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<
<<<<<<<<<<<<<<<<<]<<<[-]+[[-]>>>[-]>[-]<<<[->>+>+<<<]>>>[-<<<+>>>]<[>[-]>[-]<<<[
->>+>+<<<]>>>[-<<<+>>>]<[<<<<[-]+>->->>[-]]<[-]]<<<]<[-]+>>>>[-]>[-]<<<[->>+>+<<
<]>>>[-<<<+>>>]<[<<<<[-]>>>>[-]][-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<[-]>>>>[-]]<<<
[-]>[-]<<[->+>+<<]>>[-<<+>>]<[<<<<<<<[-]>>>>>>>[-]]<<<<<<<]